When working on Angular projects, following best practices for project organization, code structure, and architecture is crucial for maintaining scalability, readability, and maintainability. In this article, we will explore a set of best practices that can help you streamline your Angular projects and ensure a successful development process.
Best project structure bellow for any generic project
∇ app
∇ core
∇ guards
auth.guard.ts
module-import.guard.ts
no-auth.guard.ts
∇ interceptor
token.interceptor.ts
error.interceptor.ts
∇ services
service-a.service.ts
service-b.service.ts
∇ components
∇ navbar
navbar.component.html|scss|ts
∇ page-not-found
page-not-found.component.html|scss|ts
∇ constants
constant-a.ts
constant-b.ts
∇ enums
enum-a.ts
enum-b.ts
∇ models
model-a.ts
model-b.ts
∇ utils
common-functions.ts
∇ features
∇ feature-a
∇ components
∇ scoped-shared-component-a
scoped-shared-component-a.component.html|scss|ts
∇ scope-shared-component-b
scoped-shared-component-b.component.html|scss|ts
∇ pages
∇ page-a
page-a.component.html|scss|ts
∇ page-b
page-b.component.html|scss|ts
∇ models
scoped-model-a.model.ts
scoped-model-b.model.ts
∇ services
scoped-service-a.service.ts
scoped-service-b.service.ts
feature-a-routing.module.ts
feature-a.module.ts
feature-a.component.html|scss|ts
∇ shared
∇ components
∇ shared-button
shared-button.component.html|scss|ts
∇ directives
shared-directive.ts
∇ pipes
shared-pipe.ts
shared.module.ts
styles.scss
▽ styles
app-loading.scss
company-colors.scss
spinners.scss
variables.scss
▽ assets
▽ i18n
lang-a.json
lang-b.json
▽ images
image-a.svg
image-b.svg
▽ static
structure-a.json
structure-b.json
▽ icons
custom-icon-a.svg
custom-icon-b.svg
Structure explanation
1. Project Structure
A well-organized project structure is the foundation of a successful Angular application. Consider adopting the following structure:
- src: All application code goes here.
- app: The root module and core application components.
- core: Singleton services, interceptors, guards, enums, constants, and utilities.
- shared: SharedModule containing reusable components, directives, and pipes.
- features: Feature modules, each representing a distinct application feature.
- assets: Media files, fonts, and other static assets.
- styles: Global styles and mixins for consistent theming.
- environments: Configuration settings for different environments.
- app: The root module and core application components.
2. App Module
The root AppModule should be kept lean and focused. It’s the entry point of the application and should be responsible for bootstrapping the app and importing necessary modules. Avoid cluttering it with too many declarations.
3. Core Module
The CoreModule serves as the central module for root-scoped services, static components, and other singletons. It should only be loaded once at runtime and should not be lazily loaded. Use a module-import-guard to prevent re-importing it.
4. Shared Module
The SharedModule is responsible for housing reusable components, directives, and pipes. It should not have dependencies on other modules in the application and can significantly aid in reducing bundle size when using lazy loading.
Standalone Component
From angular 14 new feature introduced in angular where we don’t need to create a share module to reuse the components Read more on standalone component
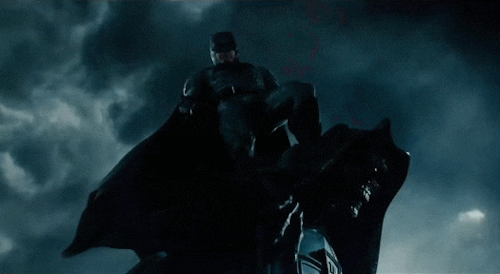
Standalone component
5. Feature Modules
Feature modules encapsulate specific functionality of your application. Each feature module should be in its own folder and should depend on the SharedModule for reusability. Consider lazy loading feature modules to improve application performance.
6. Lazy Loading
Lazy loading modules can significantly improve the initial load time of your application. Use lazy loading for feature modules that aren’t required immediately on application startup.
7. Styles and Theming
Organize your styles into separate files based on their functionality. Use mixins and CSS functions to encapsulate styles. Consider theming your application for a consistent and appealing user experience.
8. State Management
Choose an appropriate state management solution based on the complexity of your application. Options include NgRx, RxJS, and other third-party libraries. Follow established patterns like Flux or Redux for better state management. Must to read about state management here.
9. Testing
Write comprehensive unit tests and end-to-end tests using tools like Jasmine, Karma, and Protractor. Adopt test-driven development (TDD) practices to ensure code quality and prevent regressions.
10. Documentation
Maintain clear and concise documentation for your Angular project. Use tools like Compodoc to generate comprehensive documentation for your components, modules, and services.
Conclusion
By adhering to these best practices, you can create well-structured, maintainable, and scalable Angular projects. Keep in mind that every project is unique, so feel free to adapt these practices to suit your specific needs. Following these guidelines will contribute to a smoother development process and a successful Angular application.
Remember, it’s important to write an original article that reflects your own insights and experiences. If you have any questions or need assistance with specific sections, feel free to ask!